Part Two:
In part two we will cover some more fundamental aspects of the Ethereum blockchain such as wallets, transactions and gas. While giving you a brief overview of the similarities and differences of centralised applications vs decentralised applications, and what kind of development environment should be used along with some key tools for smart contract and decentralised application development.
If you have just joined the series please see part one to start from the begininng https://medium.com/bitfwd/ethereum-development-crash-course-part-one-327dee16878b
More Ethereum 🐰🕳
Article one covered some of the main key points behind Ethereum’s blockchain such as nodes and miners, forks, protocol networks, hash functions and merkle trees. This was to give you an insight to some of the low-level details of what is happening on the Ethereum Blockchain.
Don’t forget to keep what was learned in the first article for future reference within the development world of crypto, because as was stated most of the infrastructure behind public blockchains will use the same functionalities and primitives as described.
Now in part two, it’s time to start looking at more high level things that we need to know about Ethereum to become a developer within the ecosystem. Once again this will all be accountable for the basics within the crypto space so please try as hard as you to take it all in and remember. For this knowledge is very useful when speaking to a colleague, networking at events and even for a social tea or coffee with a friend.
Accounts/Wallets 💰
An account and wallet are pretty much the same thing when it boils down to the initial use case of them. They both keep a record of our transactions, balances, tokens and nonce. The difference being is that accounts are stored on the blockchain as data and wallets can come in a couple of different variants like cold and hot wallets.
Cold wallet — A wallet that is offline usually like a hardware wallet such as a Ledger Nano S or the Trezor, and also paper wallets.
Hot Wallet — A hot wallet should be considered as any wallet that is constantly online or reachable by some sort of connection, like exchange wallets Bittrex, Poloniex, Binance, mobile wallets, Metamask and desktop wallets like Exodus or MyCrypto.
Accounts on the other hand come in two forms: one is contract account and the other is EOA (externally owned account) which is a made up of two unique cryptographic keys like we discussed in article one, a public key and private key. The EOA stores the state balance of the key pair, all of the transactions that the key pair has made, who the receivers of the transaction are and how many transactions the account has made. A contract account will only store the contract balance and contract storage (i.e code, state variables, and functions)
Use the following commands to set up an exeternally owned account with the go-etheruem node.
PLEASE NOTE: The command “geth account new” does NOT require you to have a node running, you can use the command in a empty terminal.
geth account new - Blank terminal
Once you have executed the command you will be prompted to enter a password for that account.
Your new account is locked with a password. Please give a password. Don't forget this password! Passphrase: Repeat Passphrase: Address: {0x0000000000000000000000000000000000000000}
Make sure to write down your password somewhere along with the account it is linked with, usually the first and last 4–5 digits of the public key is enough to keep track of your addresses.
To set up a new EAO while you have a node that is running or syncing up with the blockchain.
Please use the following
The attach commands should be used in a fresh terminal and will give you a javascript console that will be connected to your node.
geth attach - to attach to the running node when using main net.
geth --testnet attach - if you're node is connected to a test net.
Then use the command below with your password as a parameter.
> personal.newAccount("Password")
"0x8d471a8ff59343c70780564abb29d1dac0d166c3"
How to get a list of your accounts and their balance
eth.accounts - for a list of your accounts.
eth.getBalance("0x8d471a8ff59343c70780564abb29d1dac0d166c3")
eth.getBalance will give you the balance in Wei.
web3.fromWei(eth.getBalance("0x8d471a8ff59343c70780564abb29d1dac0d166c3")
web3.fromWei - will give you the balance in ETHER.
Transactions 🏦
There are two ways you can send a transaction over the Ethereum blockchain either to another person’s EAO or to a contract address.
When sending ETH to another account all you will need the public key of the EOA you are wanting to transact with along with some ETH to pay for gas for the transaction to get stored on the blockchain. (Little more of gas in a minute)
Use the following commands in your console to send a transaction
eth.sendTransaction({from: eth.accounts[0], to: "0x0000000000000000000000000000000000000000", value: web3.fromWei(0.1, 'ether'), gas: 210000})
this will send a transaction from the first account that is stored on your node. Accounts are saved in a memory array and start at '0' if you wish to send a transaction from an account that is not the your first account, please iterate through the array with eth.accounts[1], eth.accounts[2] and so on.
NOTICE: we use 'web3.fromWei' when specifying the value of the transaction. This is because by default the value of a transaction is set in 'Wei' the lowest denomination of ether. Ether is set to 10^18 (10 with 18 decimal places) so if try to send 0.1 without using web3.fromWei you will receive an error from the console.
If you have done that CONGRATULATIONS! 👏
That’s possibly your first transaction sent to the Ethereum blockchain using a developer environment. But wait! How do you get the data of the transaction to see if it was successful. Once the transaction is sent you should receive some information on the blockchain that will look like

This is your transaction being added to the blockchain with the hash function returning the digest of your transaction which is the “fullhash” or “transaction hash”.
To retrieve the data of the transaction please use
eth.getTransaction("0xe4b76f63ebd7055cc958df80f40b82a3de24a8e37efaef53211deb99541f2c34")
To return
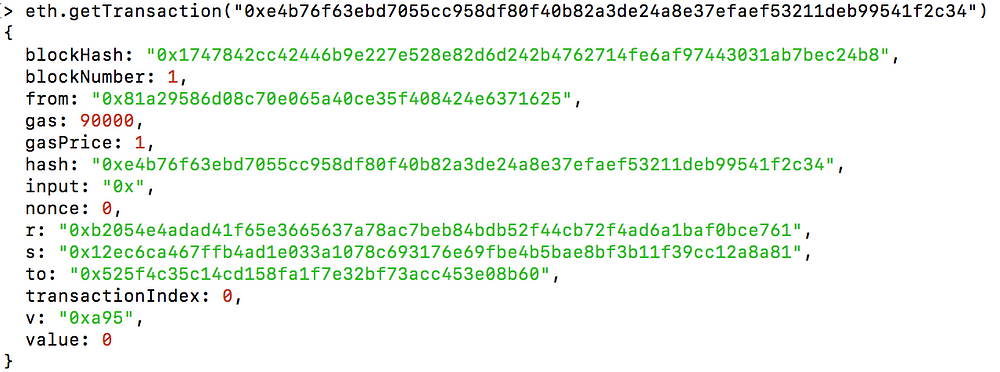
Here in the transaction data you see we have
blockHash - The hash of the block that transaction got mined in.
blockNumber - What block it is within the chain.
from - The 'from' account.
gas - The amount of gas the transaction used.
gasPrice - The price you are willing to pay for your transaction to be mined onto the network. Usual price on mainnet is around 21-40 gwei.
hash - The hash on the transaction.
input - Any data that gets added with the tx, parameters etc.
nonce - The number of the tx coming out of that account.
r, s and v - 'r' and 's' are the outputs of a ECDSA signature and 'v' is the recovery id.
to - The public key of the receiver of the transaction.
transactionIndex - Index number of tx in that block.
value - Amount of ether being sent with the transaction.
NOTE: That the transaction hash is a concatenation of all the above spun through a hash function and disburst as a hexidecimal digest (i.e transactionHash)
Great! Now you have the transaction will all the details regarding that particular hash, but how do you know if the transaction has been confirmed and executed without any errors.
Please use the following command to verify the transaction
eth.getTransactionReceipt("0xe4b76f63ebd7055cc958df80f40b82a3de24a8e37efaef53211deb99541f2c34")
To return
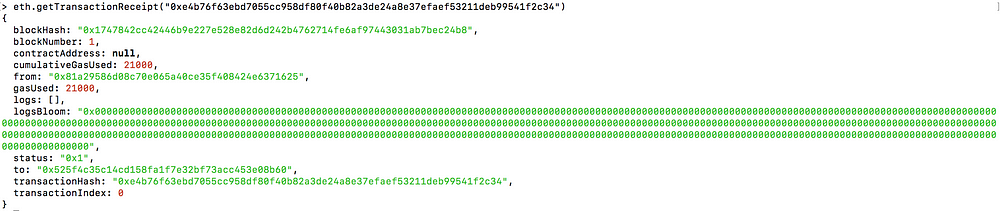
Here we have the transaction receipt which includes components such as
blockHash - The hash of the block that transaction got mined in.
blockNumber - What block it is within the chain.
contractAddress - If transaction is sent to a contract, the address with reside here.
cumulativeGasUsed - The amount of gas used in the transaction.
from - The public key of the senders account.
gasUsed - Amount of gas used in the transaction.
logs - Events that are called during a transaction execution are stored here.
logsBloom - The hexidecimal value of the event logs will be stored here.
status - If '0x0' transaction has failed. If '0x1' transaction has been executed successfully.
to - The public key of the recipients account.
transactionHash - The hash of the transaction.
transactionIndex - The index number of the tx in that block.
NOTE: These components are part of the 'transaction receipt' and only some will be included in with the transaction hash.
Awesome! You have now checked all the data from a transaction hash and have verified that the transaction was a success.
PLEASE NOTE: This was only a wallet to wallet transaction. When sending a transaction to a contract account you will need the address of the contract, some ETH in your account for the gas fee and you must also specify the name of the function you wish to call from the contract with the function parameters if needed. (We will cover transacting with a contract in a later article)
Gas ⚠️
Now it is time for probably one of the most important aspects of Ethereum and that is gas, Without gas the Ethereum blockchain would not work. Gas is literally what it says on the tin GAS. Much like the gas that fuels your car, the heating to your home or the liquid in your camping stove, gas powers the Ethereum blockchain.
Gas in Ethereum pays for the computational work being done by the EVM and the miners to process transactions and compile bytecode. Every transaction in Ethereum must have some gas sent along with it for it to be picked up by the miners of the network. If you send a transaction without gas it will just sit in the cloud of the network forever or unless you get a miner wanting to mine blocks and complete calculations for free.
You may have noticed that when you send a transaction across the network you will have to specify the gas price and gas limit.
Gas — Is the unit used to measure the fees required for a particular computation. Gas is measured in ‘Wei’
Gas Price — Is the amount of gas per unit you are willing to pay for the computation to be executed. This is mesured in ‘Gwei’ NOT ‘Wei’
Gas Limit — Is the maximum amount of gas in ‘Wei’ that you’re willing to pay.
1 Wei = 0.000000000000000001 ether.
1 Gwei = 0.000000000100000000 ether.
Example: Let’s say you want to send a transaction with a gas limit of 25,000 and a gas price of 10. This will mean that you will have to pay 25,000wei x 10gwei = 250,000wei which will be 0.00025 ETH. Currently around 10c USD to process this transaction on the main Ethereum blockchain (3/5/2018).
Any remaining gas that is left over after the transaction is completed gets refunded to the sender of the transaction. Also if the sender of the transaction does not have enough ETH in their account to pay for the transaction or not enough gas was selected for use during execution the transaction will fail. And all funds except the already consumed gas will be transferred back to the sender’s account.
Miners of the network will be the ones who collect the gas from all the transactions and contract creations, they mine into a block. Miners also have to power to manipulate the gas price of the network by only accepting transactions that have a particular gas price like 20Gwei or above. This in return allows the miners to dictate what the going rate for conversion is on executable transactions. When the network is experiencing low volumes of traction the miners will generally pump the gas price and not accept any low fees because they want to make the most out of the power comsumption being taken in by the mining rig. But on the other hand when the network is loaded with traffic the miners will generally lower their expectations of gas prices and accept relatively low fees due to the amount of transactions happening on the network.
Please see https://ethgasstation.info/index.php for the latest gas prices and information.
Centralised applications v Decentralised applications ♚♔
Traditionally applications are hosted on centralised servers such as AWS or Heroku which means they are prone to attack vectors such as DDOS attacks, SQL and data manipulation but with the decentralised nature of blockchain technologies. These vulnerabilities are wiped and applications are built with more strength and robustness to prevent hacks from happening.
The architecture of decentralised applications is very similar to centralised applications as they are first hosted on web browser which connects to a static front end that will be written is HTML, CSS and JavaScript. Then the front end will talk to an Ethereum node (Blockchain) to retrieve the data necessary for the user to interact with the application.
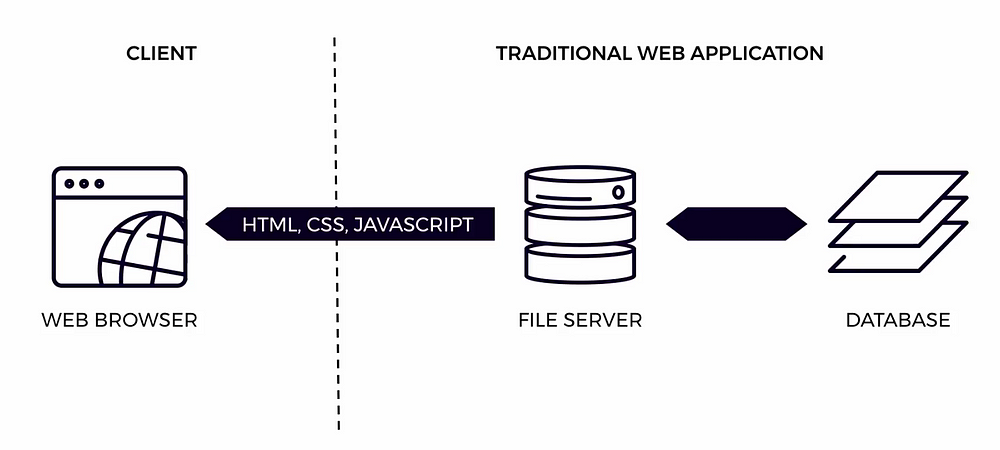
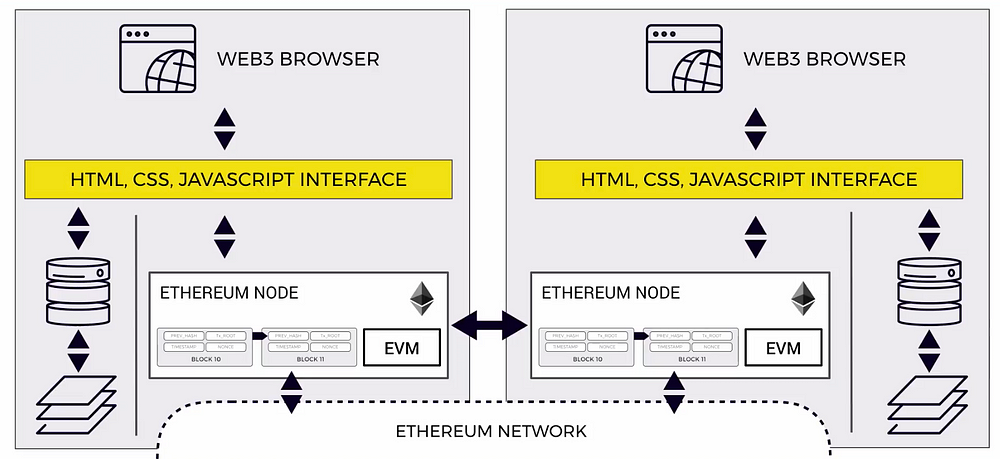
Similarities and differences ⚣⚤
Now we will just talk a quick peek at how both kinds of applications differ from eachother and how they are a like in terms of application stack, UI/UX, application logic and data.
Stack similarities ⚣
Both kinds of applications are available on mobile, tablet, laptop and pc, therefore the layout design will be relatively the same between both sorts.
Stack differences ⚤
One of the only differences in stack design architecture is the optimal plug ins for key management and identity management.
UI/UX similarities ⚣
The design for UI/UX can be achieved for both kinds of applications using common frameworks such as React, Redux, Angular, Jquery etc…
UI/UX differences ⚤
Decentralised applications are different from the traditional way of app development due to the need for web3.js to interface with the blockchain via a RPC. https://en.wikipedia.org/wiki/Remote_procedure_call
Application logic similarities ⚣
Most of the standard frameworks for business and application logic can be used for both parties, some include Trailblazer, Zend, Entity etc…
Application logic differences ⚤
Logic that needs to be in a trustless environment needs to be programmed on to the blockchain.
Data similarities ⚣
You can use traditional data modelling in both circumstances such as relational models, network models, document models, and entity-attribute-value models.
Data differences ⚤
The logic and data of a decentralised application must be progammed via a smart contract that is deployed on to the blockchain.
D-APP development enviroments 🦄
In the last part of this article we will start to look at what kind of development environments are best suited to decentralised application and smart contract development. Of course one of the first things you will need to start your venture is a text editor such as Atom, Sublime, Eclipse or Visual Studios. There is also a web browser based IDE called remix which is fantastic when developing smart contracts. If im completely honest remix is one of the best tools you can you use for Ethereum development due to the functionality it holds. You are able to write code, deploy your contract to a local blockchain, the main net or a hosted port, it even offers you a debugging tool and analyst of your contract. Along with syntax warning and errors, remix also allows you to interact with your smart contract through the IDE when deployed locally.
Some other tools such as Truffle, Embark, DappTools, Dappsys and Mythril should also be considered as very valuable to your environment as these offer you different kinds of frameworks and testing modules to apply to your application when buidling. The most commonly used is Truffles closely followed by Embark and DappTools but just for the purpose of this post we will stick to working with Truffles.
Please download Truffles framework from https://truffleframework.com. We will be using truffle in subsequent parts of the tutorial.
Credits: Connor Wiseman